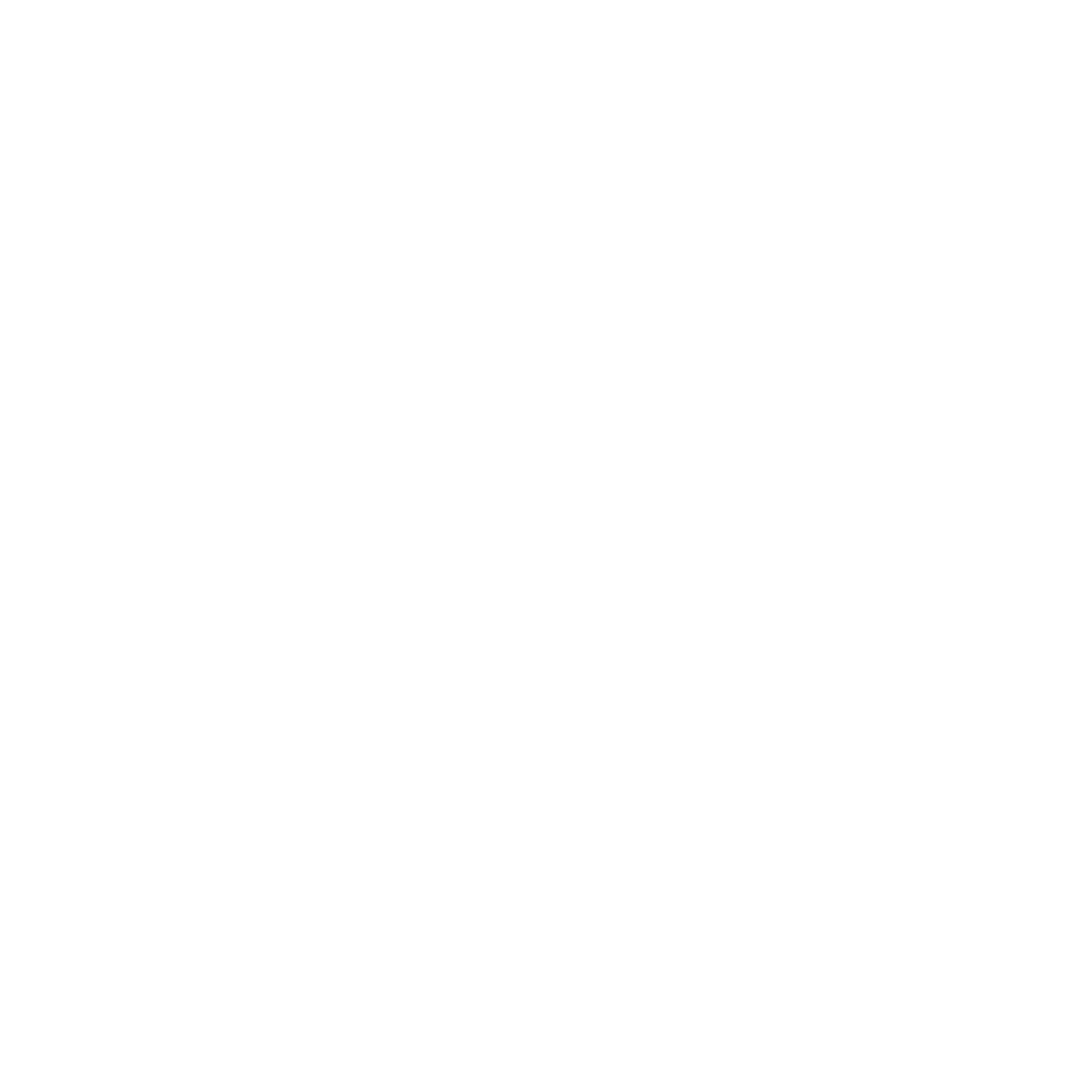
#include<stdio.h>
struct AAA {int a[10]; int n;};
struct AAA x;
void main(void)
{
int i;
int b[6]={20,35,46,18,24,52};
x.n=6;
for(i=0; i<x.n; i++) x.a[i]=b[i];
x.a[x.n]=37; x.n++;
for(i=0; i<x.n; i++) printf("%d ",x.a[i]);
printf("\n");
}
请输入运行结果:
struct Student {char numb[10]; int grade;};
#define N 20 //设N为20
{
struct Student List[N];
void Input(struct Student a[], int n) {
int i;
for(i=0; i<n; i++) {
printf("输入第%d个学生的记录的学号和成绩:",i+1);
scanf("%s %d",a[i].numb, &a[i].grade);
}
}
void Output(struct Student a[], int n) {
int i;
for(i=0; i<n; i++)
printf("%-10s %d\n", a[i].numb,a[i].grade);
}
void Sort(struct Student a[], int n) {
int i,j,k;
struct Student x;
for(i=1; i<n; i++) {
k=i-1;
for(j=i; j<n; j++)
if(a[j].grade[k].grade) k=j;
x=a[i-1]; a[i-1]=a[k]; a[k]=x;
}
printf("排序完成!\n");
}
void main(void) {
int n;
printf("输入学生记录的个数: ");
scanf("%d",&n);
printf("输入学生记录:\n"); Input(List,n); //输入n个学生记录
printf("输出学生记录:\n"); Output(List,n); //输出n个学生记录
printf("按成绩排序学生记录,"); Sort(List,n);
printf("输出排序后的学生记录:\n"); Output(List,n);
}
请输入运行结果:
#include<stdio.h>
#include<stdio.h>
struct IntNode { //结点类型定义
int data;
struct IntNode* next;
};
struct IntNode* createList();
void travList(struct IntNode* f);
int CountList(struct IntNode* f);
void main() {
struct IntNode *p1;
printf("根据键盘输入建立p1单链表: \n");
p1=createList();
printf("遍历所建立的p1单链表: \n");
travList(p1);
printf("链表中的结点个数: %d\n", CountList(p1));
struct IntNode* createList() {
int x;
int n=sizeof(struct IntNode);
struct IntNode *f, *p;
f=p=malloc; //建立附加结点
printf("输入一批整数(-1止)\n");
while(1) {
scanf("%d",&x);
if(x==-1) break;
p=p->next=malloc; //向表尾插入一个新结点
p->data=x;
}
p->next=NULL;
return f->next;
}
void travList(struct IntNode* f){
while(f) {
printf("%d ",f->data);
f=f->next;
}
printf("\n");
}
int CountList(struct IntNode* f)
{ //统计链表f的长度
int c=0; //用c作为统计变量
while(f) {
c++;
f=f->next;
}
return c;
} // CountList(p1)返回5
请输入运行结果: